Decrypting an Encoded Message
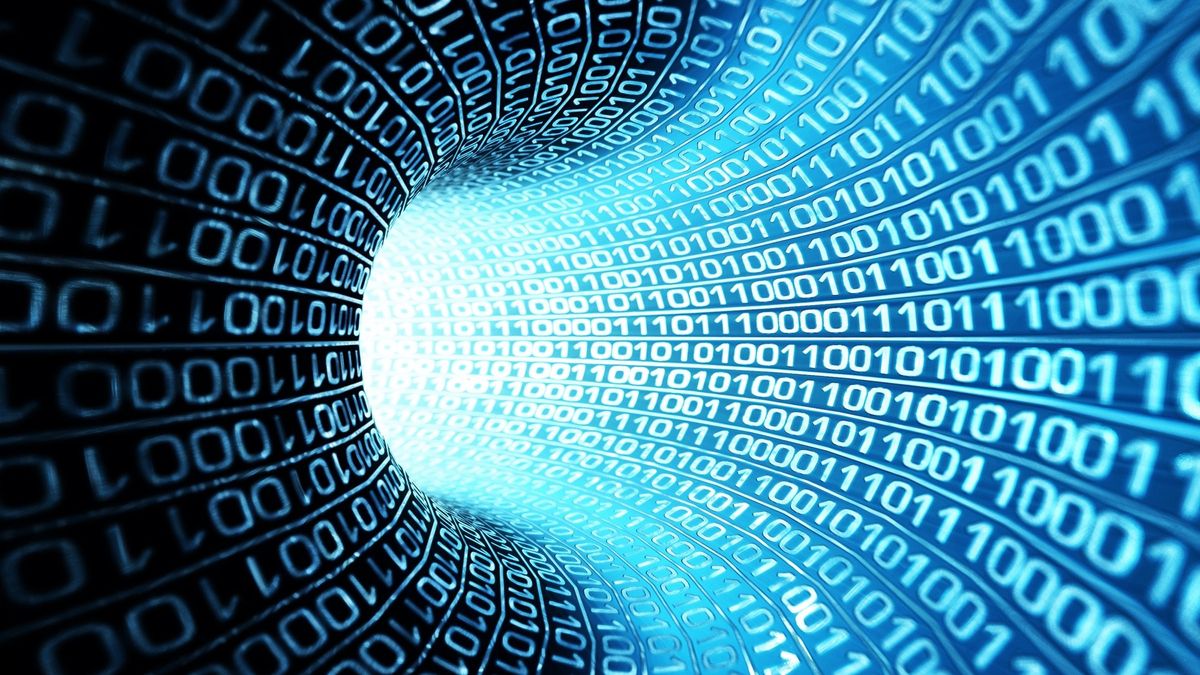
Challenge Overview:
This challenge on HackThisSite is about decoding a message that is "encrypted" using a custom encryption algorithm. The requestor for the challenge asks.
Decrypt a heavily encoded message from a CEO trying to bribe ecological inspectors investigating water pollution issues. Help environmentalists uncover corporations plotting to profit from the destruction of mother nature!
Challenge Details:
Website: www.hackthissite.org
Section: Realistic Challenge 6
The following resources were provided:
- A string of numbers seperated by periods. It's belived to be encrypted usign the fake EXCryption algorithm. A sample of the encrypted blob is shown below.
.296.294.255.268.313.278.311.270.290.305.322.252.276.286.301.305.264.301.251.269.274.311.304. 230.280.264.327.301.301.265.287.285.306.265.282.319.235.262.278.249.239.284.237.249.289.250. 282.240.256.287.303.310.314.242.302.289.268.315.264.293.261.298.310.242.253.299.278.272.333.
The breakdown:
The challenge identifies the algorithm used to “encrypt” the text as XEncryption. They provide you a form that can be used to encrypt text. I ran several specifically chosen combinations through this form. One thing you may notice is that the same input and password produce different outputs every time.
Reading below this line may spoil the challenge. Read below at your own risk.
The first thing that I noticed is that the 3 numbers it produces for each character of plain text are separated by periods and these three groups looked like they may sum up to the same value for any given input. To test this I ran several iterations of different inputs and noticed that sure enough given the same inputs the output always summed to the same value. I then tried just ‘a’ as an input then ‘b’ as an input and then ‘c’ as an input. If given with the same password the sum of the output was incremented by one each time.
My solution:
Warning: Spoiler alert! A solution is given below
My first attempted solution was to then split the input and sum each group of 3. Then build a table with the frequency each value showed up and the min and max values. I was going to look at the frequency each value appeared and compare to know distributions in the English language but decided to try something even easier first which was to assume the lowest value that would be used if this was ASCII would be the new line character which has a decimal value of 10. So I took the minimum value I found and subtracted 10 from it and assumed this was the password sum. I then went back through the blocks and subtracted this value from them and printed the ASCII value of each. This decoded to the correct value for this challenge.
The Source Code:
"""
Notes about this challenge.
Encryption/Encoding is done using an algorithm called
XECryption which after some inspection appears to simply
combine the ascii values of the plaintext character with
the sum of the ascii values of the password characters.
Because of this the encrypted content should have a common
component that contains the sum of the password characters
plus the ascii value of the character encoded. If we make
the assumption that the plaintext is an email message than
we can assume to find A-Z, a-z, 0-9, punctuation and spaces.
ASCII values 32-126 possible.
Possible approach #1
Sum up each of the 3 value blocks. Analyze the frequency.
look for the two most common ones and assume the most common
is e and the next is a. Subtract the ascii values for a and e
from their blocks and assume that is the password component,
subtract that from the remaining blocks and see if we have a
plaintext message.
"""
encrypted_content = ( ".296.294.255.268.313.278.311.270.290.305.322.252.276.286.301.305.264.301.251.269"
".274.311.304.230.280.264.327.301.301.265.287.285.306.265.282.319.235.262.278.249.239.284.237"
".249.289.250.282.240.256.287.303.310.314.242.302.289.268.315.264.293.261.298.310.242.253.299"
".278.272.333.272.295.306.276.317.286.250.272.272.274.282.308.262.285.326.321.285.270.270.241"
".283.305.319.246.263.311.299.295.315.263.304.279.286.286.299.282.285.289.298.277.292.296.282"
".267.245.304.322.252.265.313.288.310.281.272.266.243.285.309.295.269.295.308.275.316.267.283"
".311.300.252.270.318.288.266.276.252.313.280.288.258.272.329.321.291.271.279.250.265.261.293"
".319.309.303.260.266.291.237.299.286.293.279.267.320.290.265.308.278.239.277.314.300.253.274"
".309.289.280.279.302.307.317.252.261.291.311.268.262.329.312.271.294.291.291.281.282.292.288"
".240.248.306.277.298.295.267.312.284.265.294.321.260.293.310.300.307.263.304.297.276.262.291"
".241.284.312.277.276.265.323.280.257.257.303.320.255.291.292.290.270.267.345.264.291.312.295"
".269.297.280.290.224.308.313.240.308.311.247.284.311.268.289.266.316.299.269.299.298.265.298"
".262.260.337.320.285.265.273.307.297.282.287.225.302.277.288.284.310.278.255.263.276.283.322"
".273.300.264.302.312.289.262.236.278.280.286.292.298.296.313.258.300.280.300.260.274.329.288"
".272.316.256.259.279.297.296.283.273.286.320.287.313.272.301.311.260.302.261.304.280.264.328"
".259.259.347.245.291.258.289.270.300.301.318.251.305.278.290.311.280.281.293.313.259.300.262"
".315.263.319.285.282.297.283.290.293.280.237.234.323.289.305.279.314.274.291.309.273.294.249"
".283.262.271.286.310.305.306.261.298.282.282.307.287.285.305.297.275.306.280.292.291.284.301"
".278.293.296.277.301.281.274.315.281.254.251.289.313.307.244.256.302.301.317.305.239.316.274"
".277.296.269.305.301.279.287.317.284.277.305.298.264.304.286.273.275.293.309.286.282.240.287"
".239.268.269.267.315.311.292.270.271.272.336.282.237.275.316.306.239.305.314.240.296.306.270"
".247.245.302.317.316.241.291.310.266.274.274.313.288.262.319.280.276.238.297.295.287.285.288"
".301.272.275.247.305.292.286.272.310.291.301.322.256.315.298.263.281.276.237.294.284.296.284"
".302.273.298.287.298.301.265.305.270.315.278.283.302.287.263.270.345.258.270.266.302.309.262"
".260.277.327.263.277.254.283.276.239.272.264.276.279.264.267.298.264.244.245.273.292.289.273"
".248.259.263.288.290.294.210.288.268.311.318.312.242.285.293.216.262.276.340.292.299.275.259"
".293.311.234.266.294.278.307.286.267.307.285.269.310.288.274.270.326.273.276.311.304.267.302"
".318.265.299.263.283.248.257.314.288.321.321.236.284.283.227.320.312.246.261.289.316.288.263"
".312.241.265.288.298.286.287.274.306.279.276.289.307.303.293.281.298.317.252.312.283.278.263"
".304.305.258.266.270.294.286.293.290.291.291.258.254.282.282.283.313.268.282.316.310.299.254"
".264.234.296.270.265.326.288.292.293.321.305.250.320.299.253.270.296.297.298.266.312.234.273"
".287.309.286.278.269.279.316.284.276.234.293.255.267.242.253.318.270.246.278.292.285.282.314"
".266.292.286.263.313.249.290.255.289.264.292.301.299.278.291.292.225.250.261.283.303.262.264"
".264.303.299.297.274.288.267.293.316.320.317.233.303.258.302.271.283.323.247.279.268.312.269"
".297.313.280.280.273.266.332.276.313.284.281.316.279.290.273.313.308.305.260.302.306.273.234"
".279.281.284.298.278.259.290.314.275.264.339.293.322.266.261.296.306.277.275.311.284.270.318"
".259.249.286.292.301.285.280.303.283.287.299.277.273.293.228.311.283.272.304.292.277.271.306"
".302.278.298.300.287.281.309.243.272.279.282.300.291.295.284.285.252.291.251.285.283.245.250"
".252.318.298.277.235.288.259.263.278.274.307.261.260.350.250.288.256.282.316.261.285.295.292"
".300.298.264.245.241.308.301.261.253.289.264.267.300.262.248.287.257.266.275.287.297.320.287"
".264.279.297.232.231.256.288.243.252.277.274.245.256.253.229.290.263.305.278.260.294.312.283"
".301.275.276.299.297.312.275.282.294.272.228.302.324.257.261.286.326.280.283.316.294.254.258"
".275.264.236.240.277.255.231.258.286.242.277.253.296.290.250.314.320.239.292.313.261.294.261"
".317.273.285.236.292.282.271.264.297.300.272.308.299.300.269.301.269.317.284.286.262.315.276"
".279.328.269.254.252.232.272.268.309.273.264.296.305.272.267.291.324.302.297.268.268.263.298"
".300.261.312.241.254.299.280.263.292.260.301.311.317.297.248.314.272.293.298.281.298.276.311"
".291.297.318.261.274.300.293.297.267.295.261.275.334.289.238.267.289.283.257.300.262.304.311"
".278.274.265.261.345.301.296.270.273.299.289.274.272.313.282.268.320.287.320.270" )
if __name__ == "__main__":
min = 1000
max = 0
blocks = []
distribution = {}
# Break the encrypted text into chunks
chunks = encrypted_content.split('.')
# Remove empty entries
chunks = list(filter(None, chunks))
# Combine chunks into blocks by summing every 3 and build a distribution table
for i in range(0, len(chunks), 3):
val = int(chunks[i]) + int(chunks[i+1]) + int(chunks[i+2])
if (val < min):
min = val
if (val > max):
max = val
blocks.append(val)
if val in distribution:
distribution[val] += 1
else:
distribution[val] = 1
# LF & CR should be two lowest numbered values. Subtract 10 (value of LF) from the smallest value.
#This should be the sum of the password characters.
password_sum = min - 10
plain_text = []
for c in blocks:
plain_text.append(chr(c - password_sum))
print("".join(plain_text))
The challenge that I wrote about here can be found on https://www.hackthissite.org/
Note: You must join the site to see the challenges.
*Note: This post was migrated from my old blog and has an original post date of December 22, 2016.